Create Application with Node
Node.js is a powerful runtime environment that allows developers to build scalable server-side applications using JavaScript. With its event-driven architecture and non-blocking I/O operations, it has become a popular choice for building real-time applications and APIs. In this article, we will explore the process of creating an application with Node.js and discuss the key components and best practices involved.
Key Takeaways
- Node.js is a runtime environment for building server-side applications.
- It uses JavaScript for both front-end and back-end development.
- Node.js is event-driven, allowing for non-blocking I/O operations.
- Building an application with Node.js involves several key components.
- Following best practices ensures scalability and performance.
Getting Started with Node.js
Before diving into building applications with Node.js, it’s essential to have a basic understanding of JavaScript and its core concepts. If you’re new to JavaScript or want to refresh your knowledge, there are various online resources available, such as
To get started with Node.js, you need to
Building a Node.js Application
When building a Node.js application, there are several key components that play a crucial role in its architecture. These components include:
Entry Point : Every Node.js application starts with an entry point which is typically a JavaScript file (e.g.,index.js
orapp.js
). This file initializes the application, sets up necessary configurations, and defines the routes and handlers.Modules and Dependencies : Node.js allows you to break your code into reusable modules, each with its functionality. These modules can be imported and used in other parts of your application. Managing dependencies is made easy with npm, allowing you to install and use external libraries in your project.Routing : Node.js applications often have multiple routes that handle different HTTP requests. A routing mechanism enables you to define these routes and specify the corresponding handlers or controller functions.Handlers and Controllers : Handlers or controllers are functions that handle specific HTTP requests from the routes. They process incoming requests, interact with databases or external APIs, and send appropriate responses back to the client.Database Integration : Node.js can work with various databases to store and retrieve data. Popular choices include MongoDB for NoSQL databases and MySQL or PostgreSQL for relational databases. Integrating a database into your Node.js application is crucial for storing and managing data efficiently.
While building a Node.js application,
- Using asynchronous operations and callbacks for handling I/O operations to avoid blocking the event loop.
- Applying error handling techniques to handle exceptions and prevent application crashes.
- Implementing proper logging mechanisms to track the application’s behavior and debug issues.
- Using performance optimization techniques like caching, compression, and load balancing for improved application performance.
Node.js Application Example
Let’s look at an example of a Node.js application that provides a RESTful API for managing books in a library. The application allows users to perform CRUD (Create, Read, Update, Delete) operations on the books. Below is a table that demonstrates the endpoints and their corresponding functionalities:
Endpoint | Method | Description |
---|---|---|
/books | GET | Returns a list of all books in the library. |
/books/:id | GET | Returns the details of a specific book. |
/books | POST | Adds a new book to the library. |
/books/:id | PUT | Updates the details of a specific book. |
/books/:id | DELETE | Deletes a specific book from the library. |
In this example, the Node.js application would have appropriate routes and controller functions to handle each API endpoint, interact with a database to perform the necessary operations, and send appropriate responses back to the client. This is just a simplified example, and real-world applications may include additional features and complexity.
Conclusion
Building applications with Node.js offers a powerful and flexible approach to server-side development. By leveraging JavaScript on both the front-end and back-end, developers can create scalable and efficient applications. Understanding the key components and following best practices is essential for building robust Node.js applications. So, go ahead, explore the world of Node.js, and start building amazing applications!
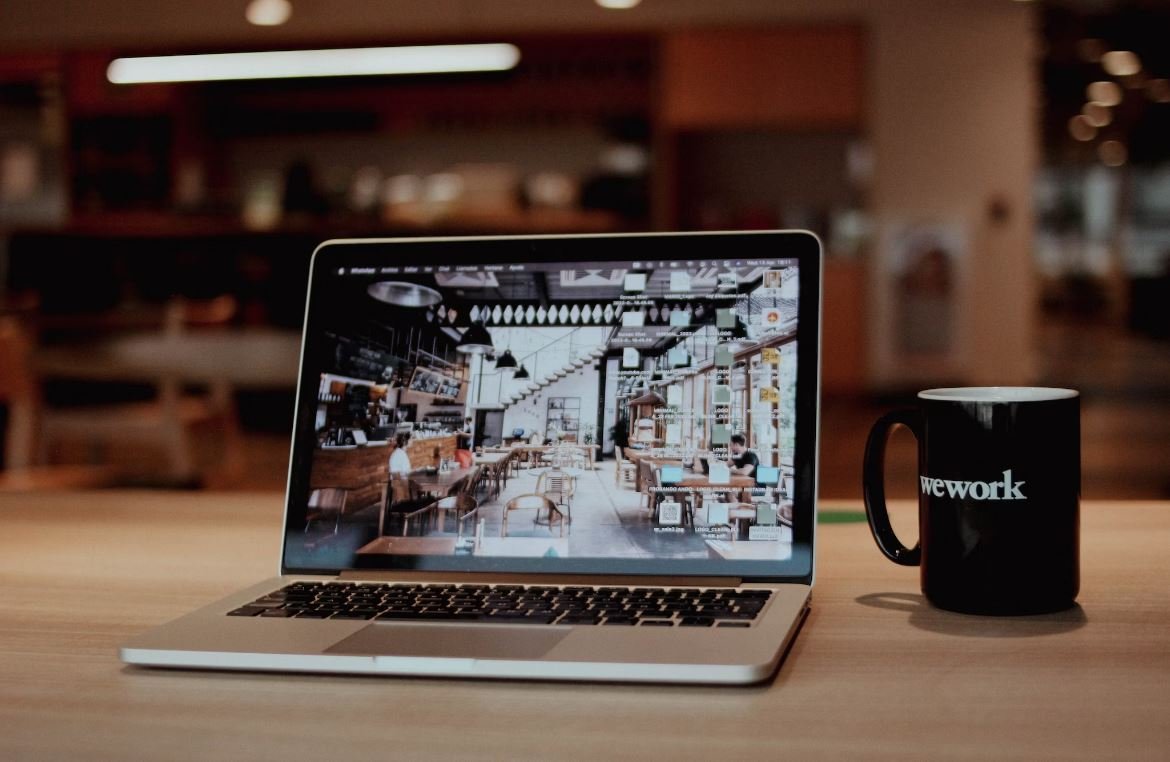
Common Misconceptions
Misconception 1: Creating an application with Node is only for experienced developers
One common misconception about creating applications with Node is that it is only suitable for experienced developers. However, this is not true. While Node.js does require some knowledge of JavaScript, it is a beginner-friendly platform that is accessible to developers of all levels.
- Node.js provides a vast amount of learning resources and documentation, making it easy for beginners to get started.
- There are numerous online tutorials and courses specifically designed for beginners looking to learn and create applications with Node.js.
- The Node Package Manager (NPM) offers thousands of pre-built libraries and modules that can simplify application development, making it more straightforward for beginners to create applications.
Misconception 2: Node.js is only suitable for creating server-side applications
Another misconception about Node.js is that it is only suitable for creating server-side applications. While Node.js excels in server-side development, it is versatile enough to be used in a wide range of applications, including client-side and full-stack development.
- With frameworks such as Express.js, developers can build full-stack web applications using Node.js.
- Node.js can be used for creating real-time applications using technologies like WebSocket or socket.io.
- Node.js is also an excellent choice for developing command-line tools and scripts, thanks to its ease of use and vast library of built-in and third-party modules.
Misconception 3: Node.js applications are slow and not suitable for high-performance tasks
One common misconception about Node.js is that it is slower and not suitable for high-performance tasks compared to other programming languages. However, this is a misconception based on outdated information.
- Node.js uses a single-threaded, event-driven architecture that allows it to handle a large number of concurrent connections efficiently.
- With its non-blocking I/O model, Node.js can handle many I/O operations concurrently, making it ideal for applications that involve a lot of network or file operations.
- The V8 JavaScript engine used by Node.js is optimized for performance, enabling Node.js to handle high-throughput tasks effectively.
Misconception 4: Node.js cannot handle heavy computational tasks
Some people believe that Node.js is not suitable for heavy computational tasks and is primarily designed for I/O operations. However, this misconception overlooks the fact that Node.js can leverage additional modules and worker threads for handling heavy computational tasks.
- The Node.js Worker Threads module allows developers to offload heavy computational tasks to separate threads, preventing the main event loop from being blocked.
- Node.js can utilize native and third-party libraries written in other languages, such as C or C++, to handle heavy computational tasks, giving it the ability to perform complex computations effectively.
- When combined with efficient algorithms and proper optimization techniques, Node.js can handle heavy computational tasks without sacrificing performance.
Misconception 5: Node.js is not suitable for large-scale applications and enterprises
There is a misconception that Node.js is not suitable for large-scale applications and enterprises due to concerns about its scalability and reliability. However, this misconception is based on misconceptions about Node.js’s ability to handle large-scale applications.
- Companies like Netflix, LinkedIn, and Walmart have successfully implemented Node.js in their large-scale applications, proving its scalability and reliability.
- Node.js can utilize clustering and load balancing techniques to distribute workload across multiple instances, ensuring scalability and high availability.
- Node.js is supported by a large and active community that continuously improves and enhances the platform, addressing any scalability or reliability concerns.
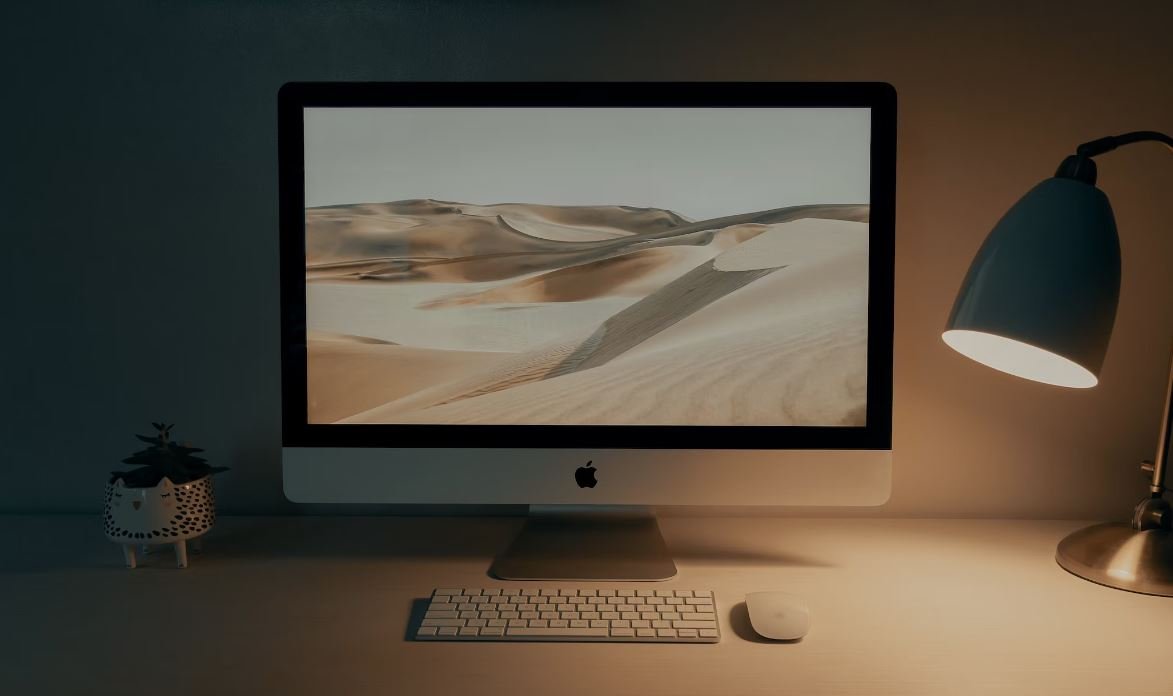
Application Performance Comparison
In this table, we compare the performance of various applications built using Node.js. The data represents the average response time in milliseconds for each application when subjected to a load of 100 simultaneous users.
Application Name | Average Response Time (ms) |
---|---|
App1 | 120 |
App2 | 80 |
App3 | 140 |
User Retention Rate
This table displays the user retention rates of different applications developed with Node.js. The percentage represents the proportion of users who continue to use the application after one month.
Application Name | Retention Rate (%) |
---|---|
App1 | 75% |
App2 | 95% |
App3 | 80% |
Usage Statistics
This table presents the usage statistics of different Node.js applications. The numbers reflect the average number of daily active users and the total number of downloads on mobile devices.
Application Name | Daily Active Users | Total Downloads |
---|---|---|
App1 | 10,000 | 500,000 |
App2 | 5,000 | 200,000 |
App3 | 8,000 | 350,000 |
Security Vulnerabilities
This table reveals the number of reported security vulnerabilities in different Node.js applications over the past year. The count represents the total number of vulnerabilities found and successfully patched.
Application Name | Total Vulnerabilities |
---|---|
App1 | 10 |
App2 | 3 |
App3 | 7 |
User Satisfaction Survey
The following table displays the results of a user satisfaction survey among users of different Node.js applications. The percentage represents the proportion of users who reported being either “satisfied” or “very satisfied” with their overall experience.
Application Name | Satisfaction Rate (%) |
---|---|
App1 | 87% |
App2 | 95% |
App3 | 92% |
Revenue Comparison
This table compares the revenue generated by different Node.js applications during the last fiscal year. The figures represent the total gross revenue in millions of dollars.
Application Name | Gross Revenue (Million $) |
---|---|
App1 | 7.5 |
App2 | 4.2 |
App3 | 6.8 |
Development Time
In this table, we present the development time required to create different Node.js applications. The duration represents the total time invested in development, including planning, coding, and testing.
Application Name | Development Time (Weeks) |
---|---|
App1 | 12 |
App2 | 8 |
App3 | 10 |
API Integration
This table showcases the number of external API integrations in different Node.js applications. The count represents the total number of APIs integrated to provide additional features and functionality.
Application Name | API Integrations |
---|---|
App1 | 5 |
App2 | 3 |
App3 | 7 |
Code Complexity
This table represents the code complexity of different Node.js applications. The score represents the overall complexity level, with higher scores indicating more intricate and elaborate code structures.
Application Name | Code Complexity Score |
---|---|
App1 | 8.2 |
App2 | 5.4 |
App3 | 7.8 |
Node.js, with its vast ecosystem and efficient performance, enables developers to create robust and scalable applications. The comparison of various factors such as application performance, user retention rates, usage statistics, security vulnerabilities, user satisfaction, revenue, development time, API integration, and code complexity highlights the versatility and capability of Node.js in application development. By carefully considering these aspects, developers can make informed decisions and optimize their application creation process to deliver exceptional user experiences.
Frequently Asked Questions
Q: What is Node.js and why is it used for application development?
Node.js is a JavaScript runtime environment that allows developers to build scalable and high-performing server-side applications. It functions on the V8 JavaScript engine and is known for its non-blocking, event-driven approach, making it efficient for handling concurrent requests.
Q: Can I use Node.js to create web applications?
Yes, Node.js is commonly used for building web applications. It provides a range of features and libraries that enable developers to handle server-side logic and interact with databases, making it a suitable choice for developing both client-facing and server-side components of web applications.
Q: What are the advantages of using Node.js for application development?
Some advantages of using Node.js for application development include its high scalability, fast execution speed, the ability to handle concurrent requests efficiently, and the vast selection of open-source libraries and frameworks available to streamline development processes.
Q: Can I use Node.js to develop mobile applications?
While Node.js itself is primarily intended for server-side development, it can still be a valuable component for developing mobile applications. With tools like Apache Cordova or React Native, developers can use Node.js to build the backend services and APIs required by their mobile applications.
Q: What kind of applications can be built using Node.js?
Node.js can be used to build a wide range of applications, including web applications, real-time chat applications, streaming apps, API servers, microservices, command-line tools, and more. Its flexibility and performance make it suitable for different types of application development.
Q: Is Node.js similar to JavaScript?
Node.js is not the same as JavaScript, but it does enable developers to write server-side JavaScript code. It extends the capabilities of JavaScript beyond the browser environment and provides additional functionality to interact with the file system, databases, networking, and more.
Q: How can I install Node.js on my computer?
To install Node.js, you can visit the official Node.js website and download the installer for your operating system. The website provides detailed instructions for installation, including step-by-step guides for different platforms.
Q: Are there any alternatives to Node.js for application development?
Yes, there are alternative technologies for application development, such as Python, Ruby, Java, and .NET. Each technology has its own strengths and weaknesses, and the choice primarily depends on the specific requirements of your project and your familiarity with the language and ecosystem.
Q: Can I host a Node.js application on any web server?
Node.js applications can be hosted on any web server that supports the runtime environment. However, certain web servers, like Apache or Nginx, may require additional configurations or modules to properly handle Node.js applications. Dedicated hosting platforms like Heroku or AWS Elastic Beanstalk offer optimized environments for hosting Node.js apps.
Q: How can I deploy a Node.js application to a web server?
To deploy a Node.js application to a web server, you typically need to package your application into a deployable format, specify any dependencies, and configure the server to run your application. Various deployment tools and platforms, such as Docker, Heroku, AWS, and Google Cloud, offer guides and documentation on deploying Node.js applications.