Make Console Application Java
Console applications are an important aspect of Java programming, allowing developers to interact with the application through text commands in the console window. In this article, we will guide you through the process of creating a console application in Java, providing you with the necessary knowledge to get started.
Key Takeaways:
- A console application is a text-based user interface that allows users to interact with a program through command-line input.
- In Java, a console application can be created by using the standard input/output streams, System.in and System.out.
- Understanding object-oriented programming concepts is crucial when developing console applications in Java.
- By following best practices and using proper exception handling, it is possible to create robust and user-friendly console applications.
Getting Started with Java Console Applications
To begin creating a console application in Java, you first need to set up your development environment. Make sure you have the Java Development Kit (JDK) installed on your machine. Then, follow these steps to get started:
- Open your preferred Integrated Development Environment (IDE) or a text editor to write your Java code.
- Create a new Java class file and give it a name that reflects the purpose of your console application.
- Import the necessary Java libraries and packages, such as
java.util.Scanner
, to handle user input. - Write the code for the functionality of your console application, making use of appropriate data structures and algorithms.
- Compile your Java code to generate a bytecode file using the javac command.
- Execute your console application using the java command, providing the name of your main class.
Remember, creating a console application in Java is just the beginning. The possibilities for extending its features and functionality are immense.
Examples of Console Applications
Console applications can serve a wide range of purposes, from simple calculators to complex data analysis tools. Here are a few examples:
Application | Description |
---|---|
Calculator | A basic console application that performs arithmetic operations based on user input. |
File Management System | A console application that allows users to organize and manipulate files and directories. |
Text-Based Game | A console application that simulates a game scenario using text-based interactions. |
Best Practices for Console Application Development in Java
When developing console applications in Java, it is essential to follow certain best practices to ensure efficient and reliable code. Consider the following:
- Modularity: Break down your application into smaller, reusable modules to enhance maintainability.
- Exception Handling: Properly handle exceptions using try-catch blocks to ensure a graceful termination of the program.
- User Input Validation: Validate user inputs and provide meaningful error messages to enhance user experience.
Remember, adhering to best practices not only improves the quality of your console application but also makes it easier for others to understand and contribute to your code.
Advantages | Disadvantages |
---|---|
Easy to develop and test. | Limited graphical capabilities. |
Quick to prototype. | Lack of interactivity. |
Lightweight and efficient. | Less visually appealing. |
Conclusion
Creating console applications in Java can open up a world of possibilities in terms of functionality and user interaction. By understanding the basic concepts and following best practices, you can develop robust and powerful console applications to suit various needs. So, embrace the text-based interface and explore the endless potential of Java console applications!
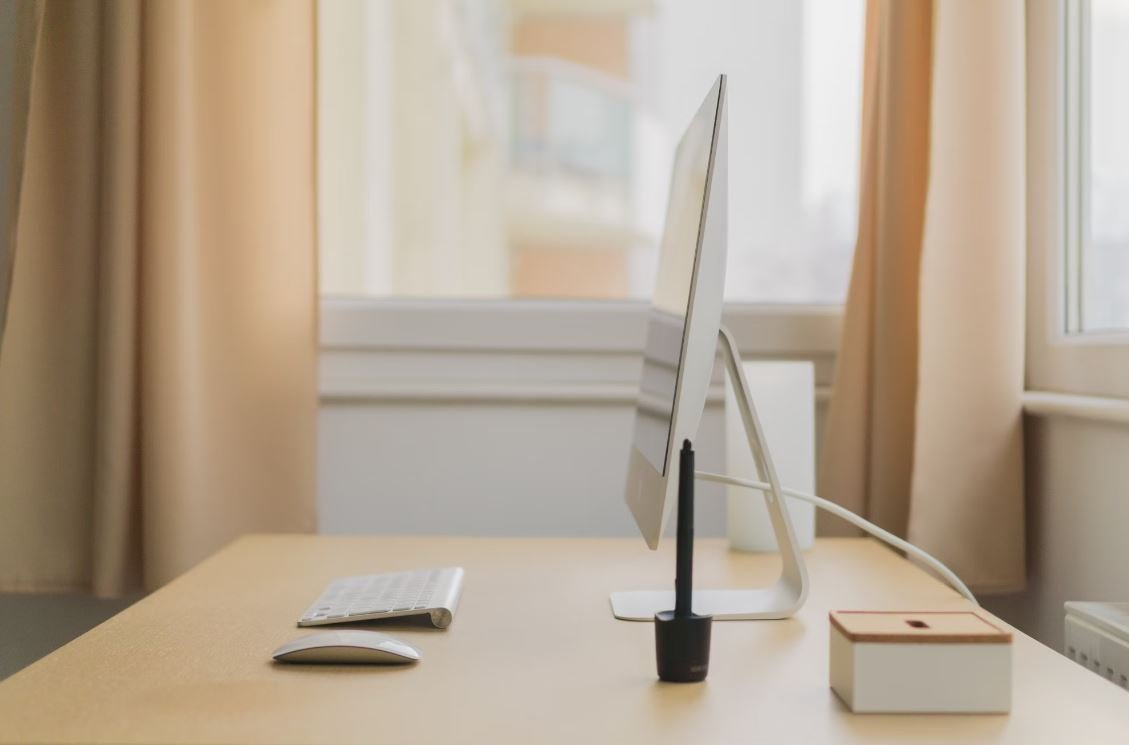
Common Misconceptions
Misconception 1: Console Applications are Outdated
- Console applications are still widely used in various industries.
- Console applications are often used for system administration tasks and batch processing.
- Console applications provide a lightweight and efficient way to perform specific tasks.
One common misconception is that console applications are outdated and have been replaced by more modern and graphical user interfaces (GUIs). However, this is not entirely true. Console applications are still widely used in various industries and have their own unique advantages. They are often utilized for system administration tasks and batch processing. For example, in the banking industry, console applications are frequently employed for automated transactions and data processing. Furthermore, console applications provide a lightweight and efficient way to perform specific tasks without the overhead of a GUI.
Misconception 2: Console Applications are Complex
- Console applications can be simple and straightforward to develop.
- Console applications focus mainly on the logic and functionality of the program.
- Console applications do not require any user interface design or graphical components.
Another misconception is that console applications are complex and difficult to develop. In reality, console applications can be simple and straightforward to create. They do not require any user interface design or graphical components, which simplifies the development process. Console applications focus mainly on the logic and functionality of the program, making them ideal for tasks that do not require a graphical interface. This simplicity allows developers to focus on the core functionality of the application without getting distracted by the complexities of GUI programming.
Misconception 3: Console Applications are Limited in Functionality
- Console applications can perform a wide range of tasks, from data manipulation to calculations.
- Console applications can interact with databases and external systems.
- Console applications can be extended and enhanced with additional libraries and frameworks.
A common misconception is that console applications are limited in functionality compared to GUI applications. However, this is not true. Console applications can perform a wide range of tasks, from simple data manipulation to complex calculations. They can interact with databases and external systems, making them suitable for handling data-centric operations. Additionally, console applications can be extended and enhanced with additional libraries and frameworks. This allows developers to leverage a vast ecosystem of tools and resources to add functionality to their console applications.
Misconception 4: Console Applications are Not User-Friendly
- Console applications can provide clear and concise instructions for users.
- Console applications can accept user input through command-line arguments or prompts.
- Console applications can display formatted output for easier readability.
Some people believe that console applications are not user-friendly because they lack the visual appeal and interactivity of GUI applications. However, console applications can still provide a user-friendly experience. They can provide clear and concise instructions for users, guiding them through the steps required to use the application. Console applications can accept user input through command-line arguments or prompts, allowing users to interact with the program. Moreover, console applications can display formatted output for easier readability, improving the overall user experience.
Misconception 5: Console Applications are Inefficient
- Console applications are lightweight and do not consume significant system resources.
- Console applications can process large amounts of data efficiently.
- Console applications can run on a wide range of devices, including low-spec hardware.
There is a misconception that console applications are inefficient compared to GUI applications. However, console applications are lightweight and do not consume significant system resources. They are designed for efficient processing of data and can handle large amounts of information without a heavy performance overhead. Furthermore, console applications can run on a wide range of devices, including low-spec hardware, making them versatile and accessible for various computing environments. These characteristics make console applications a suitable choice for tasks that prioritize efficiency and resource usage.
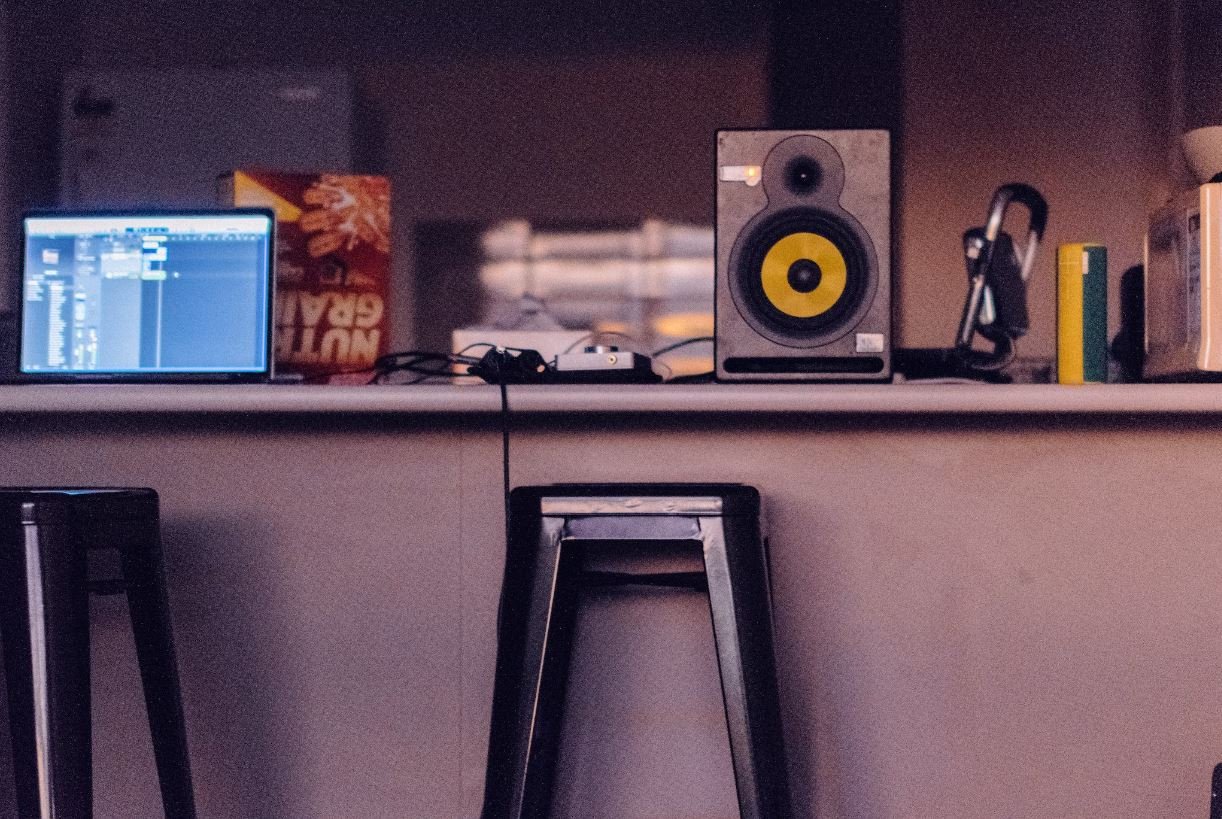
Make Console Application Java
A console application in Java is a program that runs in a command line interface, interacting directly with the user through text input and output. It provides an efficient way to perform tasks, process data, and automate various operations. In this article, we will explore ten interesting aspects of creating a console application in Java.
1. Command Line Arguments
Command line arguments provide a convenient way to pass parameters to a console application without modifying the source code. These arguments can be accessed within the Java program using the `String[] args` parameter in the main method. They enable users to customize program behavior or provide input data at runtime.
2. User Input
Function | Description |
---|---|
Scanner | Java’s Scanner class allows reading input from the console. It provides methods like `nextLine()`, `nextInt()`, etc., to retrieve user input in various data types. |
BufferedReader | BufferedReader class reads text from the character input stream. It can be used to read user input line by line through methods like `readLine()`. |
Getting user input is crucial for interactive console applications. Java provides various techniques to read and process user input efficiently. The Scanner class and BufferedReader class are commonly used for this purpose.
3. Text Output Formatting
Properly formatted text output enhances the user experience and readability of a console application. Java supports formatting options through the `System.out.printf()` method, which allows displaying formatted text with placeholders (%s, %d, etc.) and their corresponding values.
4. Exception Handling
Exception handling is essential for robust console applications. Java’s try-catch blocks enable developers to handle potential errors and exceptions gracefully. By catching and handling exceptions, we can prevent the application from exiting abruptly and provide meaningful error messages to the user.
5. File Input/Output
Console applications often need to read from or write to files. Java offers classes like FileInputStream, FileOutputStream, FileReader, and FileWriter to perform file input/output operations. These classes enable reading, writing, and manipulating files seamlessly within a console application.
6. Multithreading
Concurrency is a crucial aspect for high-performance console applications. Java’s multithreading capabilities allow running multiple tasks simultaneously, improving overall application efficiency. By utilizing threads and thread synchronization techniques, developers can make console applications more responsive and efficient.
7. External Libraries
Library | Description |
---|---|
Apache Commons CLI | Apache Commons CLI provides a convenient way to parse command line arguments, handle options, and generate usage help messages. |
jline | jline is a library that facilitates advanced console interactions, including input history, line editing, autocompletion, and more. |
Java offers a vast ecosystem of external libraries that can enhance console application development. Libraries like Apache Commons CLI and jline provide additional features and functionalities to make console applications more powerful and user-friendly.
8. Progress Indicators
Long-running console applications can benefit from progress indicators to keep users informed about the program’s state. Implementing progress bars or percentage completion indicators can enhance the user experience and make the application more engaging.
9. Interactive Menus
Interactive menus provide a convenient way for users to navigate and interact with console applications. Java’s switch-case statement or if-else branching can be utilized to create smooth and intuitive menu systems, enabling users to select different actions or options.
10. Logging
Logging plays a crucial role in debugging and error tracking within console applications. Java provides robust logging frameworks like Log4j and SLF4J, which allow developers to record program events, errors, and informational messages, aiding in application maintenance and troubleshooting.
Creating console applications in Java opens up a vast array of possibilities for developers. Whether it’s taking user inputs, handling exceptions, utilizing external libraries, or making the application visually appealing, Java provides a comprehensive set of tools to create robust and engaging console applications.
FAQ: Make Console Application Java
FAQ 1: What is a console application?
A console application, also known as a command-line application, is a program that interacts with the user through a text-based interface in the console or terminal window rather than a graphical user interface.
FAQ 2: How can I create a console application in Java?
To create a console application in Java, you need to write Java code using a text editor or an integrated development environment (IDE) such as Eclipse or IntelliJ IDEA. Save the file with a .java
extension, compile it using the Java compiler, and then run the compiled bytecode using the Java Virtual Machine (JVM).
FAQ 3: What are the advantages of using a console application?
Console applications have several advantages, including:
- Lightweight and efficient
- No need for graphical user interface development
- Easier debugging and testing
- Tighter control over program execution
FAQ 4: Can console applications have user input and output?
Yes, console applications can interact with the user by reading input from the console and displaying output. You can use the Scanner
class to read user input and the System.out.println()
method to display output in the console.
FAQ 5: How can I handle command-line arguments in a console application?
In Java, you can access command-line arguments passed to a console application through the args
parameter in the main()
method. The args
parameter is an array of strings that stores the command-line arguments.
FAQ 6: Are there any best practices for designing console applications?
Some best practices for designing console applications in Java include:
- Use clear and concise user prompts
- Handle user input validation
- Provide informative error messages
- Organize code into functions or classes for modularity
FAQ 7: How can I handle user input validation in a console application?
To validate user input in a console application, you can use conditional statements such as if
or switch
to check if the input meets specific criteria. If the input is invalid, you can display an error message and prompt the user to enter valid input.
FAQ 8: Can I use third-party libraries or frameworks in a console application?
Yes, you can use third-party libraries or frameworks in a console application, just like in any other Java application. However, keep in mind that using external dependencies may increase the complexity of your project and require additional setup.
FAQ 9: How can I handle exceptions in a console application?
To handle exceptions in a console application, you can use try-catch blocks. Place the code that might throw an exception inside the try block, and catch the exceptions in the catch block to handle or log them appropriately.
FAQ 10: How can I package and distribute a console application in Java?
To package and distribute a console application in Java, you can create a runnable JAR file using the Java Archive (JAR) tool or an IDE. The JAR file contains all the necessary class files, resources, and dependencies needed to run the application on any system with Java installed.